Vue Js Enlarger Image: zoom in and zoom out is a useful feature in web development that enables users to change the size of an image for improved viewing. Vue.js provides several options for implementing this functionality, including CSS transforms and Vue.js directives. By using CSS transforms, you can use the “scale” transform to increase or decrease the size of an image on click. Vue.js directives, on the other hand, provide a way to bind dynamic behavior to an element and can be used to create a custom directive for handling image zoom in and zoom out
How to zoom image onclick using Vue Js
- The code creates a new Vue instance and associates it with the DOM element with ID “app”.
- The data object in the Vue instance contains two properties: “url”, which is the URL of an image, and “isZoomed”, a Boolean value that determines if the image is zoomed or not.
- The methods object in the Vue instance contains a single method called “toggleZoom”. This method toggles the value of “isZoomed” between true and false.
- In the HTML template, an image element is displayed with its source URL bound to the “url” property in the Vue instance’s data.
- The “transform” style property of the image is also bound to the “isZoomed” property in the data. When “isZoomed” is true, the image is scaled to 2 times its original size, otherwise it is scaled to 1 times its original size.
- The “click” event of the image is bound to the “toggleZoom” method, so that when the image is clicked, the “isZoomed” value will be toggled and the image will zoom in or out.
Vue Js Image Zoom out or in on click | Example
<div id="app">
<img :style="{ transform: isZoomed ? 'scale(2)' : 'scale(1)' }" @click="toggleZoom" :src="url" />
</div>
<script type="module">
const app = new Vue({
el: "#app",
data() {
return {
url: 'https://www.sarkarinaukriexams.com/images/editor/1670265927Capture123243243423.PNG',
isZoomed: false
}
},
methods: {
toggleZoom() {
this.isZoomed = !this.isZoomed;
}
}
})
</script>
Output of above example
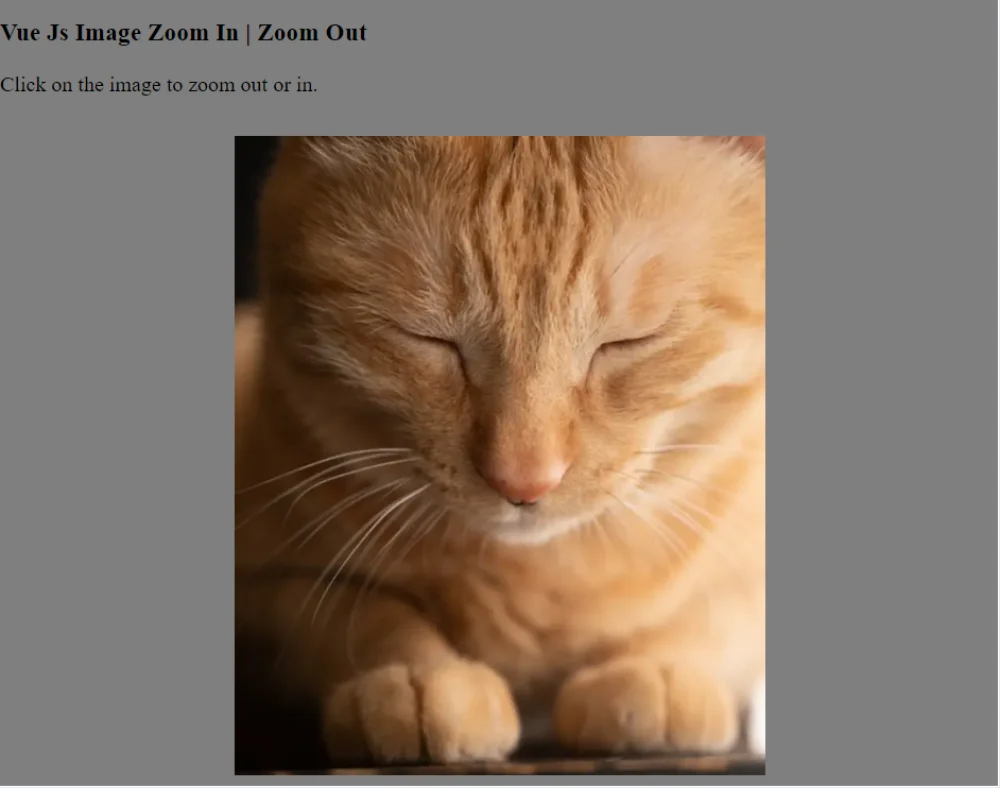